Problem Solving in Java | Basic Java Programming Solution | AskTheCode
Problem:
Rambo is not good in Mathematics. And, his Math test is happening now in which he's to solve some algebraic expressions. As the test is happening online, so Rambo asked you to help you.
You being a programmer help him to get results of algebraic expressions with the help of a Java program. Rambo has sent you the image of his test questions, help him solve them.
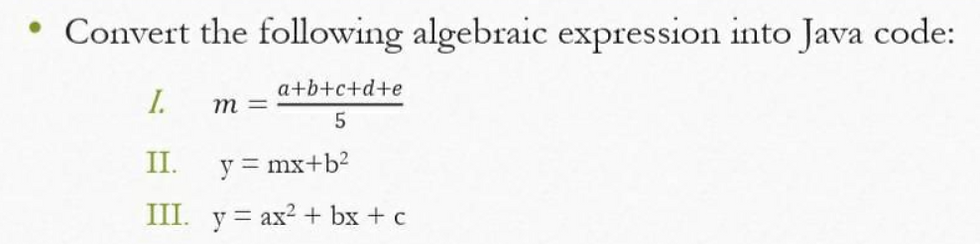
Write a Java for each of the expressions shown above in the picture.
Note: You don't need to take input from the user. He'll put all the values directly.
Solutions :
For expression m = (a + b + c + d + e) / 5
Sample Input:
a = 10, b = 20, c = 30, d = 40, e = 40
Sample Output:
28
Code:
public class Solution{
public static void main(String []args){
int a = 10, b = 20, c = 30, d = 40, e = 40;
int m = (a + b + c + d + e) / 5;
System.out.println(m);
}
}
For expression y = mx + b ^ 2
Sample Input:
m = 10, x = 5, b = 9
Sample Output:
131
Code:
public class Solution{
public static void main(String []args){
int m = 10, x = 5, b = 9;
int y = m * x + (b * b);
System.out.println(y);
}
}
For expression y = ax ^ 2 + bx + c
Sample Input:
a = 5, b = 7, c = 4, x = 8
Sample Output:
380
Code:
public class Solution{
public static void main(String []args){
int a = 5, b = 7, c = 4, x = 8;
int y = a * (x * x) + b * x + c;
System.out.println(y);
}
}
Comments