Calculate BMI in Java | AskTheCode
- Team ATC
- Mar 3, 2021
- 1 min read
Updated: Mar 9, 2021
Java program to calculate BMI and also interpret it | Java programming solution | Ask The Code
Problem:
Write a Java program that reads values for weight in kilograms and height in centimeters and prints out the BMI ( Body Mass Index). Your program should also print on screen an interpretation of the BMI. Use the following scale:
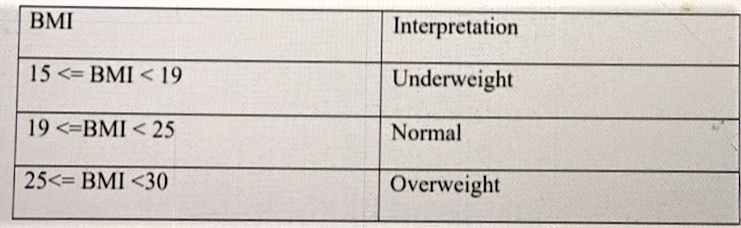
Input:
A Line contains weight (in kg) and height (in cm) separated by a space.
Output:
The first line displaying BMI up to 2 decimal points.
Next line displaying the interpretation of the BMI according to the provided scale.
Code:
import java.util.*;
public class BMI_Calculator{
/* Function for converting kg to pounds */
public static double kilogramToPound(double kilogram){
double pounds = kilogram * 2.2;
return pounds;
}
/* Function for converting cm to inches */
public static double centimetresToInches(double centimetres){
double inches = centimetres * 0.39;
return inches;
}
/* Function for calculating BMI */
public static double bmi_calc(double weight, double height){
double bmi = (weight * 703) / (height * height);
return bmi;
}
public static void main(String []args){
Scanner sc = new Scanner(System.in);
System.out.println("Enter your weight (in kg) and height (in cm) separated by a space: ");
String s = sc.nextLine();
s = s.trim();
double height = Double.valueOf(s.substring(s.indexOf(" ")+1,s.length()));
double weight = Double.valueOf(s.substring(0,s.indexOf(" ")));
weight = kilogramToPound(weight);
height = centimetresToInches(height);
double bmi = bmi_calc(weight, height);
System.out.printf("%.2f",bmi);
System.out.println();
if (bmi >= 15 && bmi < 19)
System.out.println("Underweight");
else if (bmi < 25)
System.out.println("Normal");
else if (bmi < 30)
System.out.println("Overweight");
}
}
Comments